3. Defining a DDS System in XML¶
Connector loads the definition of a DDS system from an XML configuration file that includes the definition of domains, DomainParticipants, Topics, DataReaders and DataWriters, data types and quality of service.
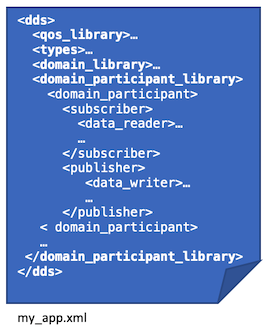
Connector uses the XML schema defined by RTI’s XML-Based Application Creation feature.
Hint
The Connext DDS C, C++, Java and .NET APIs can also load the same XML files you write for Connector.
The following table summarizes the XML tags, the DDS concepts they define, and how they are exposed in the Connector API:
XML Tag |
DDS Concept |
Connector API |
---|---|---|
|
DDS data types (the types associated with Topics) |
|
|
DDS Domain, Topic |
Defines the domain joined by a |
|
DomainParticipant |
Each |
|
Publisher and DataWriter |
Each |
|
Subscriber and DataReader |
Each |
|
Quality of service (QoS) |
Quality of service used to configure |
Hint
For an example configuration file, see ShapeExample.xml.
3.1. Data types¶
The <types>
tags defines the data types associated with the Topics to be published
or subscribed to.
The following example defines a ShapeType with four members: color
, x
, y
and shapesize
:
<types>
<struct name="ShapeType">
<member name="color" type="string" stringMaxLength="128" key="true"/>
<member name="x" type="int32"/>
<member name="y" type="int32"/>
<member name="shapesize" type="int32"/>
</struct>
</types>
Types are associated with Topics, as explained in the next section, Domain library.
Hint
You can define your types in IDL and convert them to XML with rtiddsgen.
For example: rtiddsgen -convertToXml MyTypes.idl
For more information about defining types, see Creating User Data Types with XML in the Connext DDS Core Libraries User’s Manual.
For more information about accessing the data samples, see Accessing the data.
3.2. Domain library¶
A domain library is a collection of domains. A domain specifies:
<domain_library name="MyDomainLibrary">
<domain name="MyDomain" domain_id="0">
<register_type name="ShapeType" type_ref="ShapeType"/>
<topic name="Square" register_type_ref="ShapeType"/>
<topic name="Circle" register_type_ref="ShapeType"/>
</domain>
</domain_library>
For more information about the format of a domain library, see XML-Based Application Creation: Domain Library.
3.3. Participant library¶
A DomainParticipant joins a domain and contains Publishers and Subscribers, which contain DataWriters and DataReaders, respectively.
Each Connector()
instance created by your application is associated with a
<domain_participant>, as explained in Loading a Connector.
DataWriters and DataReaders are associated with a DomainParticipant and a
Topic. In Connector, each <data_writer>
tag defines an Output()
, as described in
Writing Data (Output); and each <data_reader>
tag defines an Input()
,
as described in Reading Data (Input).
<domain_participant_library name="MyParticipantLibrary">
<domain_participant name="MyPubParticipant" domain_ref="MyDomainLibrary::MyDomain">
<publisher name="MyPublisher">
<data_writer name="MySquareWriter" topic_ref="Square" />
</publisher>
</domain_participant>
<domain_participant name="MySubParticipant" domain_ref="MyDomainLibrary::MyDomain">
<subscriber name="MySubscriber">
<data_reader name="MySquareReader" topic_ref="Square" />
</subscriber>
</domain_participant>
</domain_participant_library>
For more information about the format of a participant library, see XML-Based Application Creation: Participant Library.
3.4. Quality of service¶
All DDS entities have an associated quality of service (QoS). There are several ways to configure it.
You can define a QoS profile and make it the default. The following example configures all DataReaders and DataWriters with reliable and transient-local QoS:
<qos_library name="MyQosLibrary">
<qos_profile name="MyQosProfile" is_default_qos="true">
<datareader_qos>
<reliability>
<kind>RELIABLE_RELIABILITY_QOS</kind>
</reliability>
<durability>
<kind>TRANSIENT_LOCAL_DURABILITY_QOS</kind>
</durability>
</datareader_qos>
<datawriter_qos>
<reliability>
<kind>RELIABLE_RELIABILITY_QOS</kind>
</reliability>
<durability>
<kind>TRANSIENT_LOCAL_DURABILITY_QOS</kind>
</durability>
</datawriter_qos>
</qos_profile>
</qos_library>
You can define the QoS for each individual entity:
<domain_participant name="MyPubParticipant" domain_ref="MyDomainLibrary::MyDomain">
<domain_participant_qos> <!-- ... --> </domain_participant_qos>
<publisher name="MyPublisher">
<publisher_qos> <!-- ... --> </publisher_qos>
<data_writer name="MySquareWriter" topic_ref="Square">
<datawriter_qos>
<reliability>
<kind>RELIABLE_RELIABILITY_QOS</kind>
</reliability>
<durability>
<kind>TRANSIENT_LOCAL_DURABILITY_QOS</kind>
</durability>
</datawriter_qos>
</data_writer>
</publisher>
</domain_participant>
Or you can use profiles and override or define additional QoS policies for each entity:
<domain_participant name="MyPubParticipant" domain_ref="MyDomainLibrary::MyDomain">
<domain_participant_qos base_name="MyQosLibrary::MyQosProfile">
<!-- override or configure additional Qos policies -->
</domain_participant_qos>
<publisher name="MyPublisher">
<publisher_qos base_name="MyQosLibrary::MyQosProfile">
<!-- override or configure additional Qos policies -->
</publisher_qos>
<data_writer name="MySquareWriter" topic_ref="Square">
<datawriter_qos base_name="MyQosLibrary::MyQosProfile">
<!-- override or configure additional Qos policies -->
</datawriter_qos>
</data_writer>
</publisher>
</domain_participant>
You can also use built-in profiles and QoS snippets. For example, the following profile is equivalent to MyQosProfile above:
<qos_library name="MyQosLibrary">
<qos_profile name="MyQosProfile" is_default_qos="true">
<base_name>
<element>BuiltinQosSnippetLib::QosPolicy.Durability.TransientLocal</element>
<element>BuiltinQosSnippetLib::QosPolicy.Reliability.Reliable</element>
</base_name>
</qos_profile>
</qos_library>
You can read more in the RTI Connext DDS Core Libraries User’s Manual, Configuring QoS with XML.
3.4.1. Logging¶
Logging can be configured as explained in Configuring Logging via XML.
For example, to increase the logging verbosity from the default (ERROR) to
WARNING, define a qos_profile
with the attribute
is_default_participant_factory_profile="true"
:
<qos_profile name="Logging" is_default_participant_factory_profile="true">
<participant_factory_qos>
<logging>
<verbosity>WARNING</verbosity>
</logging>
</participant_factory_qos>
</qos_profile>